Introduction
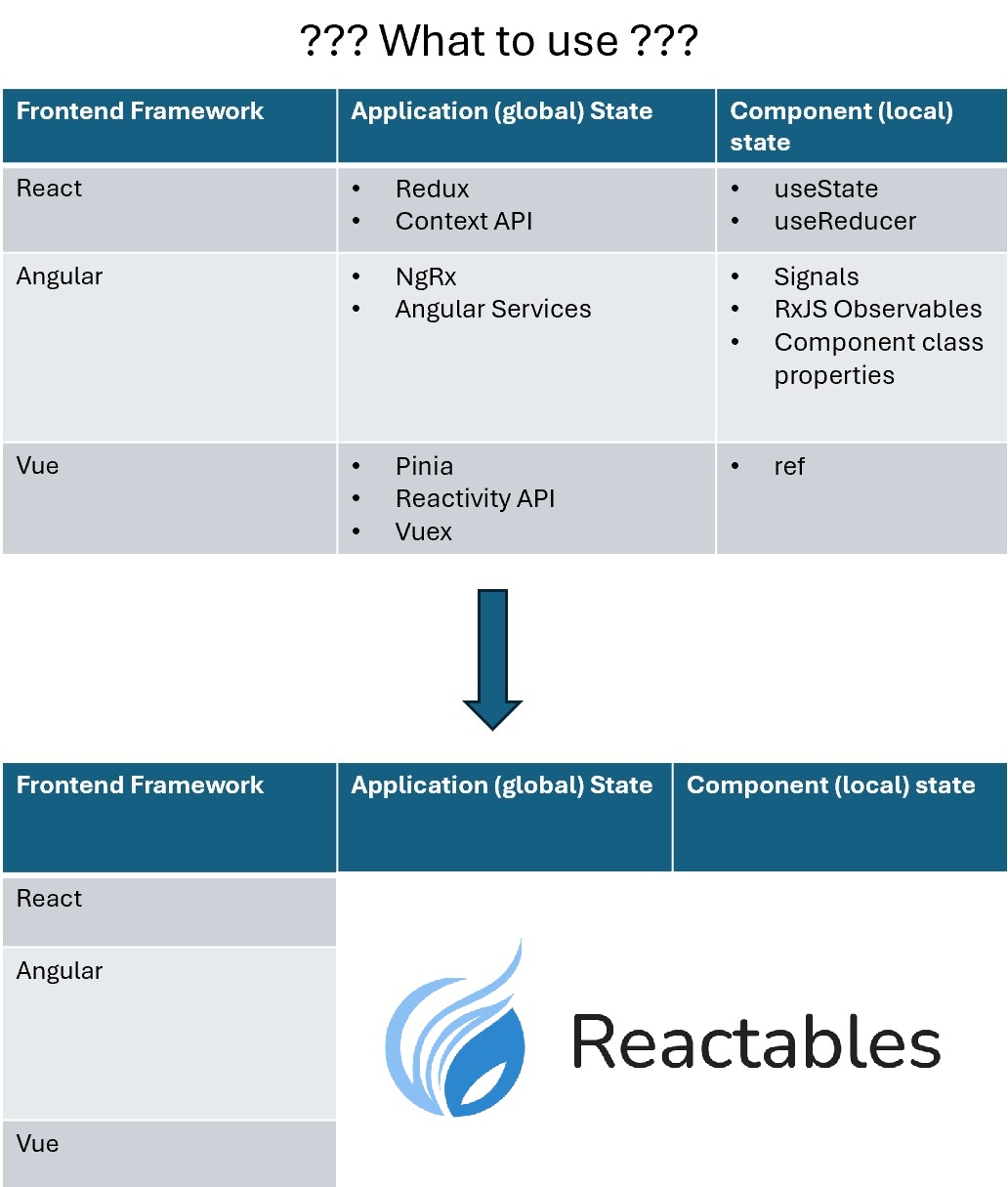

Reactables provide one core API for handling a wide range of state management use-cases.
It leverages RxJS and reactive/declarative patterns to allow more time to focus on what you want to achieve in your buisness logic and save time on how it is implemented.
Installation
Requires RxJS 6 or above. If not already installed, run npm i rxjs
npm i @reactables/core
Create your first Reactable!
import { RxBuilder } from '@reactables/core';
export const RxToggle = (initialState = false) =>
RxBuilder({
initialState,
reducers: {
toggleOn: () => true,
toggleOff: () => false,
toggle: (state) => !state,
},
});
Bind to the view!
Edit this snippet
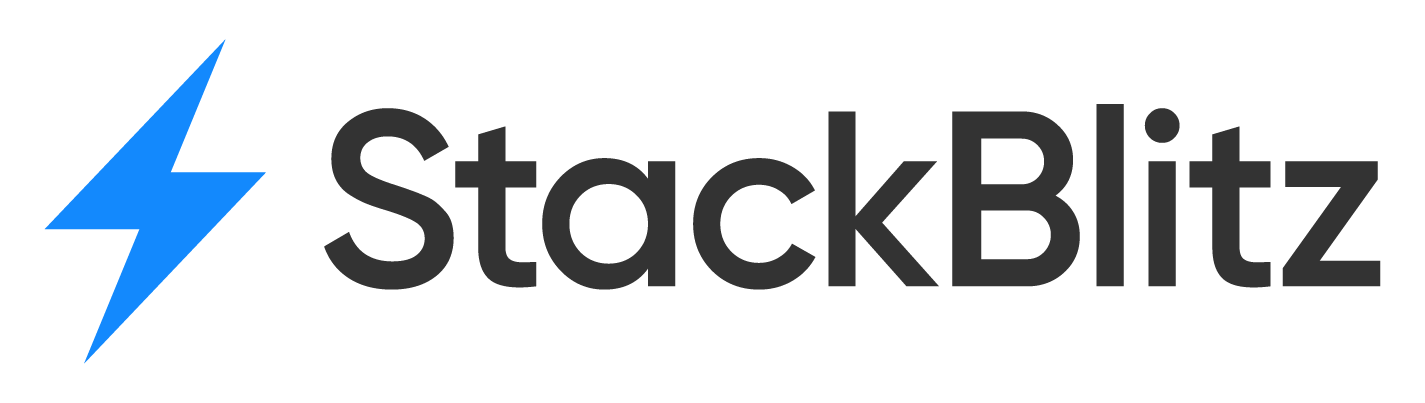
import { RxToggle } from './RxToggle';
import { useReactable } from '@reactables/react';
function App() {
const [toggleState, actions] = useReactable(RxToggle);
const { toggleOn, toggleOff, toggle } = actions;
return (
<>
<h5>Reactable Toggle</h5>
Toggle is: {toggleState ? 'On' : 'Off'}
<br />
<button onClick={toggleOn}>Toggle On</button>
<button onClick={toggleOff}>Toggle Off</button>
<button onClick={toggle}>Toggle</button>
</>
);
}
export default App;
Edit this snippet
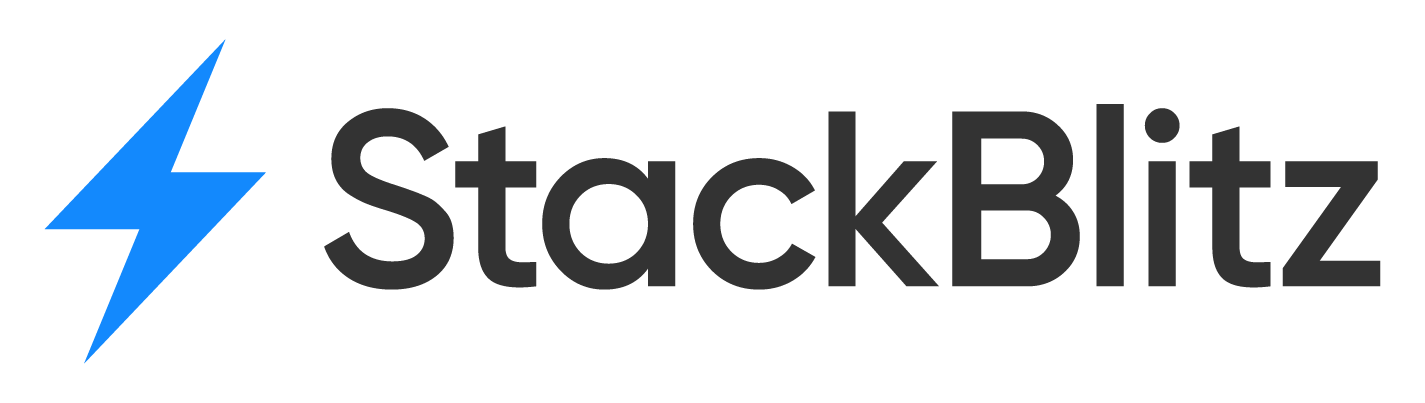
import { Component } from '@angular/core';
import { CommonModule } from '@angular/common';
import { RxToggle } from './RxToggle';
// See Reactable Directive
// at https://reactables.github.io/angular/reactable-directive
import { ReactableDirective } from './reactable.directive';
@Component({
selector: 'app-root',
standalone: true,
imports: [CommonModule, ReactableDirective],
template: `
<div *reactable="rxToggle; let state = state; let actions = actions;">
<h1>Angular Reactable Toggle: {{ state ? 'on' : 'off' }}</h1>
<button (click)="actions.toggleOn()">Toggle On </button>
<button (click)="actions.toggleOff()">Toggle Off </button>
<button (click)="actions.toggle()">Toggle </button>
</div>
`,
})
export class App {
rxToggle = RxToggle();
}
Edit this snippet
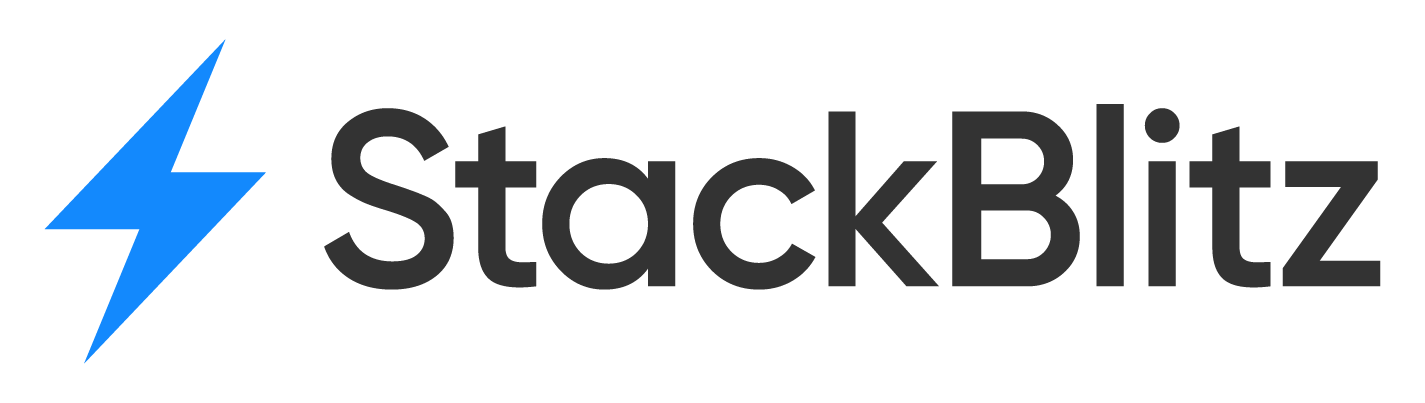
import { RxToggle } from './RxToggle';
const [state$, actions] = RxToggle();
const { toggleOn, toggleOff, toggle } = actions;
state$.subscribe((toggleState) => {
// Update the view when state changes.
document.getElementById('toggle-state')
.innerHTML = toggleState ? 'On' : 'Off';
});
// Bind click handlers
document.getElementById('toggle-on')
.addEventListener('click', toggleOn);
document.getElementById('toggle-off')
.addEventListener('click', toggleOff);
document.getElementById('toggle')
.addEventListener('click', toggle);