Core Concepts
Reactable Interface
const [state$, actions] = RxToggle();
Reactables (prefixed with Rx) provide a clean separation of concerns between state logic and presentation in your UI - enhancing testability and extensibility.
Reactables encapsulate the state logic and expose an observable which UI components can subscribe to for state changes.
For predictable state changes, the UI state can only be changed by invoking action methods provided by the reactable.
Reactable Primitive
A reactable primitive is the basic building block for modelling your state.
It can be used alone or combine with other primitives to form more complex reactables as the state of your component/feature/application grows.
Hub and Store
Internally, a reactable primitive has a hub and store. The hub dispatches actions to the store where state updates occur.
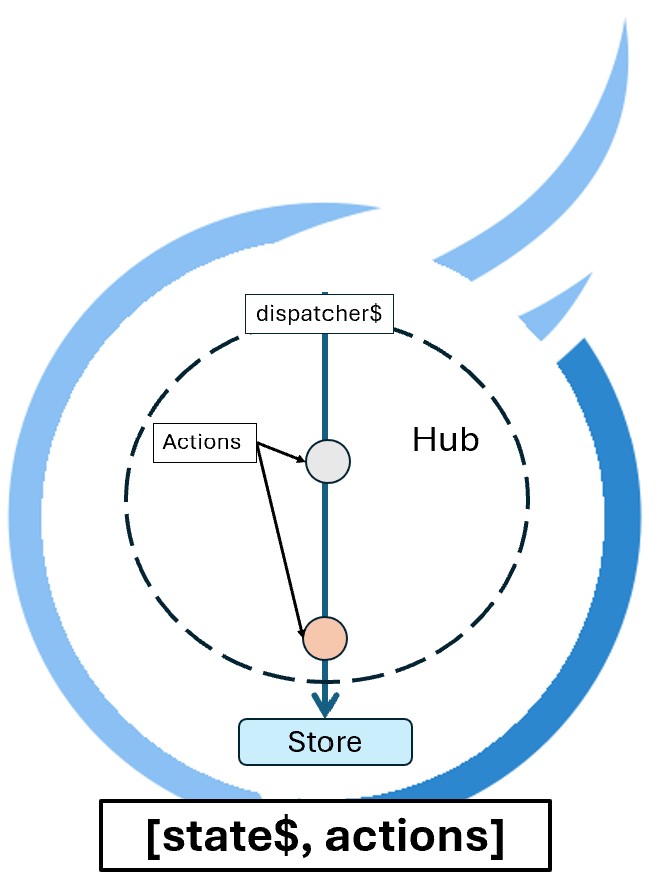
See Basic Toggle for an example.
Effects
The hub also handles side effects (i.e API requests) with effects
.
When an action is dispatched and a side effect is needed, a replayed action is sent through an effect stream to execute the side effect.
Responses are then mapped into actions and relayed to the store.
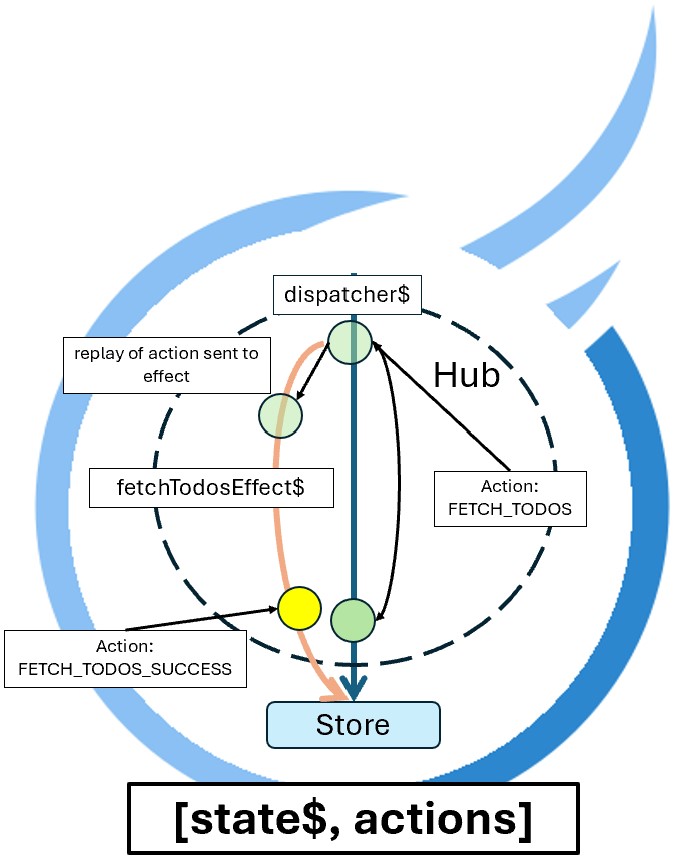
See Fetching Data with an Effect for example.
Sources
Reactables also have the ability to receive actions from any number of external sources and react to them.
Composition, Reactive Programming and Unidirectional Flow
Organizing state into slices is sensible when state grows and becomes more complex. Reactable primitives can represent these slices and through composition they can create a new reactable to handle increasing complexity.
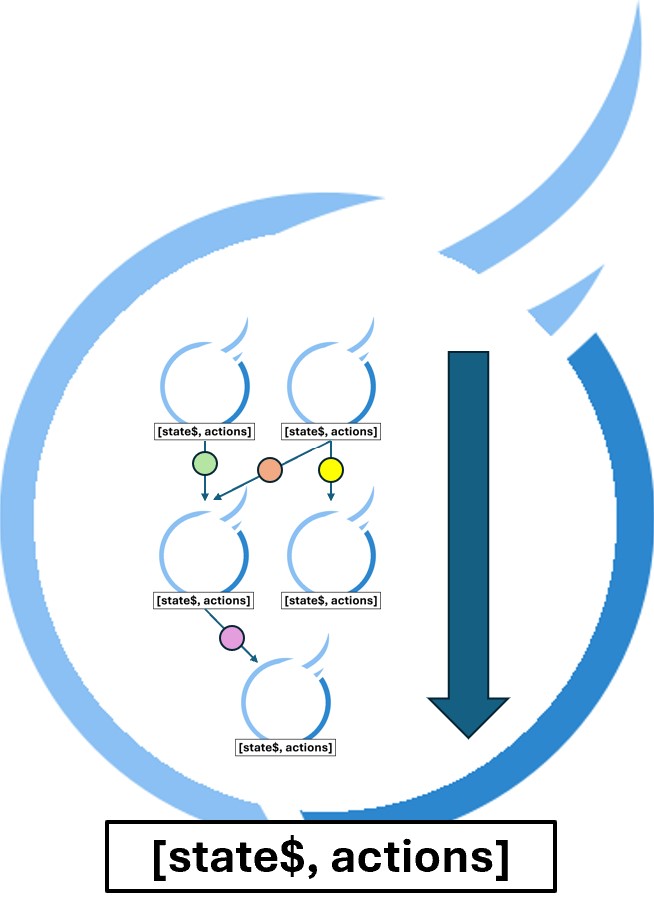
Reactables encourages a reactive programming style where a reactable’s sources of change are explicit in its declaration.
This results in a unidirectional flow of actions making state changes highly predictable.
See Composition with Reactables for example.